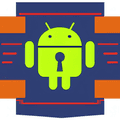
Securing Sensitive Data using Gradle Files
MONDAY JANUARY 02 2023 - 1 MIN
When developing an Android application, it's important to keep sensitive data such as API keys secure. One technique for doing this is to store the data in an external gradle file.
Storing Secrets in a Properties File
A Properties
object can be used to load key-value pairs from a file. This allows you to store sensitive data such as API keys and passwords in a separate file that can be excluded from version control.
Here's an example of how to load secrets from a secrets.properties
file:
def secretsPropertiesFile = rootProject.file("secrets.properties")
def secretProperties = new Properties()
if (secretsPropertiesFile.exists()) {
secretProperties.load(new FileInputStream(secretsPropertiesFile))
}
Using Environment Variables
If the secrets.properties file does not exist, you can use environment variables as a fallback. This is useful when building your application on a continuous integration server where you may not have access to the secrets.properties file.
Here’s an example of how to read an environment variable named API_KEY and set it as a property:
if (!secretsPropertiesFile.exists()) {
secretProperties.setProperty("API_KEY", "${System.getenv('API_KEY')}")
}
Setting Build Config Fields
Once you have loaded your secrets into a Properties object, you can use them to set build config fields. This allows you to access the values at runtime using the generated BuildConfig
class.
Here’s an example of how to set two build config fields for API_BASE_URL
and API_KEY
, using values from the previously loaded properties:
android {
defaultConfig {
buildConfigField "String", "API_BASE_URL", "\"${secretProperties['API_BASE_URL']}\""
buildConfigField "String", "API_KEY", "\"${secretProperties['API_KEY']}\""
}
}
Storing sensitive data in a separate properties file or using environment variables are effective techniques for keeping your data secure. By setting build config fields with these values, you can easily access them at runtime while keeping them out of version control.
For suggestions and queries, just contact me.