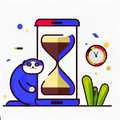
Lazy Loading Libraries in Dart
SUNDAY MAY 21 2023 - 2 MIN
When it comes to building large scale applications, efficient use of resources is one of the most important considerations. This includes not only memory usage but also network bandwidth, disk space, and CPU cycles. One way of achieving this is through lazy loading.
Lazy loading refers to loading code or resources only when they are actually needed. This approach can greatly reduce the size and complexity of an application by loading only the necessary parts, thus improving startup time, reducing network bandwidth, and reducing memory usage.
In Dart, the deferred
keyword is used to implement lazy loading of libraries. This keyword allows you to specify that a library should be loaded only when needed, rather than being loaded when the application starts up.
How to Use Deferred Loading in Dart
To use deferred loading in Dart, you need to follow a few simple steps.
-
Declare the library as
deferred
import 'package:deferred_library/deferred_library.dart' deferred as deferred_library;
-
Use the library when needed
someFunction() async { await deferred_library.loadLibrary(); deferred_library.someFunctionInLibrary(); }
In this example, the
loadLibrary
method is called before accessing any function defined in thedeferred_library
. This method loads the library if it has not already been loaded. Once the library is loaded, any function or variable defined in it can be used normally.
Advantages of Using Deferred Loading
Using deferred loading in your Dart application provides several advantages:
-
Reduced application loading time: By loading only the necessary code when it is actually needed, the application startup time can be greatly reduced.
-
Reduced memory usage: If a library is not used frequently, it is wasteful to keep it in memory all the time. Deferred loading ensures that the library is loaded only when needed, thus reducing memory usage.
-
Better network bandwidth usage: If the library is loaded lazily, the initial download size of the application can be reduced, resulting in faster downloads and better network bandwidth usage.
Conclusion
Lazy loading is a crucial technique for optimizing resource usage in large-scale applications. Dart has a built-in capability to load libraries lazily using the deferred
keyword. This allows you to load only the necessary parts of the application, thus reducing memory usage, network bandwidth, and startup time. By using this technique judiciously, you can greatly improve the performance of your Dart application.
For suggestions and queries, just contact me.